Can Web scraping and data extraction apps like Firecrawl , be used in Dialogflow?
ChatGPT
Thought for 26 seconds
Certainly! Web scraping and data extraction tools like Firecrawl can be integrated with Dialogflow to enhance your conversational agent's capabilities. By using these tools in conjunction with Dialogflow's fulfillment webhook, you can fetch real-time data from websites and provide dynamic responses to user queries.
Below is a comprehensive guide on how to use web scraping tools like Firecrawl with Dialogflow, along with important considerations to ensure legal and ethical compliance.
Important Considerations Before You Begin
1. Legal and Ethical Compliance
-
Terms of Service: Always check the target website's terms of service to ensure that web scraping is permitted. Unauthorized scraping may violate legal agreements and lead to legal consequences.
-
Data Privacy: Be cautious when scraping personal or sensitive data. Comply with data protection laws like GDPR or HIPAA if applicable.
-
Respect Robots.txt: Check the website's
robots.txt
file to see if scraping is disallowed. -
Ethical Use: Use the scraped data responsibly and ethically, ensuring it benefits users without harming others.
2. Technical Considerations
-
Latency: Web scraping can introduce delays due to network requests and data processing. Optimize your scraping code for efficiency.
-
Reliability: Websites may change their structure, causing your scraper to break. Implement error handling and regular maintenance.
-
Performance Impact: Scraping can consume significant resources. Ensure your backend can handle the load without affecting the user experience.
Steps to Integrate Web Scraping Tools Like Firecrawl with Dialogflow
1. Set Up Your Web Scraping Backend
Since Dialogflow fulfillment requires a backend server to process requests, you'll need to set up an environment where you can run your web scraping code.
a. Choose a Backend Environment
-
Programming Language: Select a language compatible with both Dialogflow and your web scraping tool (e.g., Python, Node.js).
-
Hosting Platform: Options include Google Cloud Functions, AWS Lambda, Firebase Functions, or your own server.
b. Install Firecrawl or Similar Tools
-
Firecrawl Integration: If Firecrawl provides an API or SDK, install it in your backend environment.
-
Alternative Libraries:
-
Python:
BeautifulSoup
,Scrapy
,Requests
. -
Node.js:
Puppeteer
,Cheerio
,Axios
.
-
c. Implement the Scraping Logic
-
Define Scraping Functions: Write functions to scrape the required data from target websites.
-
Example in Python Using
Requests
andBeautifulSoup
:pythonimport requests from bs4 import BeautifulSoup def scrape_data(url): response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Extract specific data data = soup.find('div', class_='data-class').text return data
-
Example in Node.js Using
Axios
andCheerio
:javascriptconst axios = require('axios'); const cheerio = require('cheerio'); async function scrapeData(url) { const { data } = await axios.get(url); const $ = cheerio.load(data); // Extract specific data const result = $('div.data-class').text(); return result; }
2. Create a Fulfillment Webhook for Dialogflow
a. Develop the Webhook Endpoint
-
Webhook Setup:
-
The webhook should accept POST requests from Dialogflow.
-
Parse the request to extract intent and parameters.
-
-
Handle Requests:
-
Identify the intent and call the appropriate scraping function.
-
Process the scraped data and formulate a response.
-
-
Send Response Back:
-
Format the response as per Dialogflow's webhook response format.
-
b. Deploy the Webhook
-
Hosting:
-
Deploy your webhook on a platform that supports HTTPS (required by Dialogflow).
-
Examples: Google Cloud Functions, AWS Lambda with API Gateway, Heroku.
-
-
Security:
-
Use secure protocols.
-
Optionally, implement authentication to prevent unauthorized access.
-
3. Configure Dialogflow to Use the Fulfillment Webhook
a. Enable Webhook in Dialogflow Console
-
Access Fulfillment Settings:
-
In the Dialogflow console, navigate to Fulfillment in the left menu.
-
-
Enable Webhook:
-
Toggle the Webhook switch to Enabled.
-
-
Set Webhook URL:
-
Enter the HTTPS URL of your deployed webhook.
-
b. Associate Intents with the Webhook
-
Edit Intents:
-
For each intent that requires web scraping, open the intent settings.
-
-
Enable Webhook Call:
-
Scroll to the Fulfillment section and enable Webhook call for this intent.
-
4. Test Your Integration
-
Use Dialogflow Console:
-
Enter test queries in the Try it now panel.
-
-
Verify Responses:
-
Ensure the agent responds with data fetched from your scraper.
-
-
Debug Issues:
-
Check logs in your backend environment for errors or exceptions.
-
Example Scenario: Creating a Medical Information Agent
Suppose you're building an agentic doctor that provides medical definitions or drug information by scraping a reputable medical website.
1. Implement Scraping Function
-
Target Website:
-
Use a medical website that allows scraping or provides an API (e.g., MedlinePlus).
-
-
Scraping Logic:
-
Extract definitions or explanations based on user queries.
-
2. Develop Fulfillment Webhook
-
Handle Medical Queries:
-
Extract the medical term from the user's input using entities.
-
-
Fetch Data:
-
Scrape the definition or information related to the term.
-
-
Formulate Response:
-
Return the information to the user with appropriate disclaimers.
-
3. Configure Intent in Dialogflow
-
Training Phrases:
-
"What is diabetes?"
-
"Tell me about ibuprofen."
-
-
Entities:
-
@medical_term: Custom entity containing medical terms.
-
-
Fulfillment:
-
Enable webhook call for the intent.
-
Best Practices
1. Use APIs When Available
-
Preference for APIs:
-
APIs are more stable, legal, and efficient.
-
-
Example APIs:
-
MedlinePlus Connect API: For health information.
-
OpenFDA API: For drug and medical device data.
-
2. Implement Caching
-
Reduce Load:
-
Cache frequent responses to minimize scraping frequency.
-
-
Improve Performance:
-
Faster response times enhance user experience.
-
3. Error Handling and User Feedback
-
Graceful Failures:
-
Inform the user if data cannot be retrieved.
-
-
Fallback Responses:
-
Provide alternative options or suggest rephrasing.
-
4. Compliance and Disclaimers
-
Medical Disclaimer:
-
Include statements that the information is not professional medical advice.
-
-
Data Usage:
-
Be transparent about how user data is used and stored.
-
5. Regular Maintenance
-
Update Scraping Logic:
-
Websites may change; update your code accordingly.
-
-
Monitor Performance:
-
Use logging and monitoring tools to track your agent's performance.
-
Alternative Approach: Using Dialogflow with Knowledge Bases
If web scraping seems complex or risky, consider using Dialogflow's Knowledge Connectors:
-
Knowledge Bases:
-
Import documents or FAQs into Dialogflow.
-
-
Automatic Responses:
-
Dialogflow can automatically match user queries to knowledge articles.
-
-
Ease of Use:
-
Simplifies the process without needing a backend.
-
Sample Code Snippet for Webhook (Node.js with Express)
javascript
const express = require('express'); const app = express(); const axios = require('axios'); const { WebhookClient } = require('dialogflow-fulfillment'); app.post('/webhook', express.json(), (req, res) => { const agent = new WebhookClient({ request: req, response: res }); async function handleMedicalQuery(agent) { const term = agent.parameters.medical_term; try { const data = await scrapeMedicalInfo(term); agent.add(`Here is what I found about ${term}: ${data}`); } catch (error) { console.error(error); agent.add("I'm sorry, I couldn't retrieve that information right now."); } } let intentMap = new Map(); intentMap.set('MedicalInfoIntent', handleMedicalQuery); agent.handleRequest(intentMap); }); async function scrapeMedicalInfo(term) { // Implement your scraping logic here const response = await axios.get(`https://example.com/search?q=${term}`); // Parse response and extract data const data = parseData(response.data); return data; } function parseData(html) { // Use Cheerio or similar library to parse HTML // Extract the desired information return extractedData; } app.listen(process.env.PORT || 8080, () => { console.log('Server is running...'); });
Conclusion
Integrating web scraping tools like Firecrawl with Dialogflow allows you to provide dynamic, real-time information to users. By setting up a backend webhook that handles scraping and connecting it to your Dialogflow agent, you can enhance your agent's functionality significantly.
However, it's crucial to proceed with caution:
-
Ensure Legal Compliance: Always have permission to scrape the target websites.
-
Prioritize User Experience: Optimize for performance to prevent delays.
-
Maintain Ethical Standards: Use the data responsibly and include necessary disclaimers.
Additional Resources
-
Dialogflow Documentation:
-
Web Scraping Libraries:
-
APIs for Medical Data:
-
Legal Guidelines:
Feel free to ask if you need further assistance with integrating web scraping tools into Dialogflow or if you have other questions!
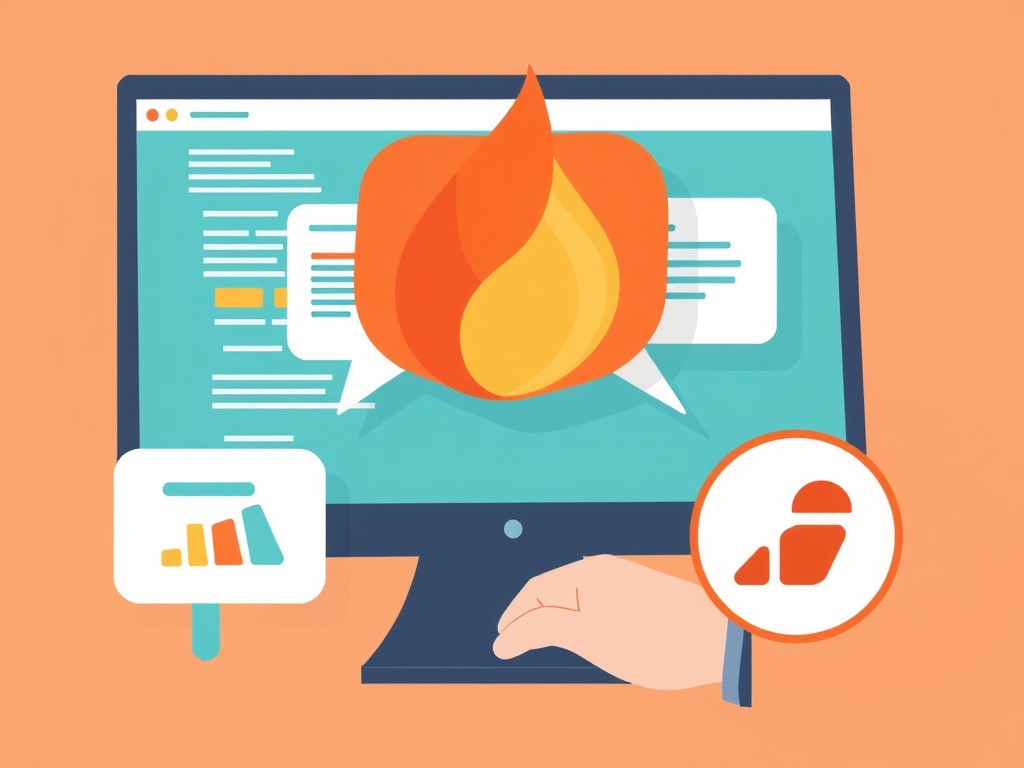